1697. Checking Existence of Edge Length Limited Paths
给你一个 n 个点组成的无向图边集 edgeList ,其中 edgeList[i] = [ui, vi, disi] 表示点 ui 和点 vi 之间有一条长度为 disi 的边。请注意,两个点之间可能有 超过一条边 。给你一个查询数组queries ,其中 queries[j] = [pj, qj, limitj] ,你的任务是对于每个查询 queries[j] ,判断是否存在从 pj 到 qj 的路径,且这条路径上的每一条边都 严格小于 limitj 。请你返回一个 布尔数组 answer ,其中 answer.length == queries.length ,当 queries[j] 的查询结果为 true 时, answer 第 j 个值为 true ,否则为 false 。
1 | 输入:n = 3, edgeList = [[0,1,2],[1,2,4],[2,0,8],[1,0,16]], queries = [[0,1,2],[0,2,5]] |
方法一:离线算法+并查集。比赛中没时间做,此题要用并查集。
在线算法,可以用来处理数据流。算法不需要一次性地把所有的 query 都收集到再处理。大家也可以想象成:把这个算法直接部署到线上,尽管在线上可能又产生了很多新的 query,也不影响,算法照常运行。
离线算法则不同。离线算法需要把所有的信息都收集到,才能运行。处理当前 query 的计算过程,可能需要使用之后 query 的信息。
以排序算法为例,插入排序算法是一种在线算法。因为可以把插入排序算法的待排序数组看做是一个数据流。插入排序算法顺次把每一个数据插入到当前排好序数组部分的正确位置。在排序过程中,即使后面源源不断来新的数据也不怕,整个算法照常进行。
选择排序算法则是一种离线算法。因为选择排序算法一上来要找到整个数组中最小的元素;然后找第二小元素;以此类推。这就要求不能再有新的数据了。因为刚找到最小元素,再来的新数据中有更小的元素,之前的计算就不正确了。
按照queries的limit排序,这样我们只需要查看当前的边是否联通来知道是否这条路径上的每条边都小于limit。
1 | def distanceLimitedPathsExist(self, n: int, edgeList: List[List[int]], queries: List[List[int]]) -> List[bool]: |
1319. Number of Operations to Make Network Connected
最少需要多少步可以将所有的服务器相连。
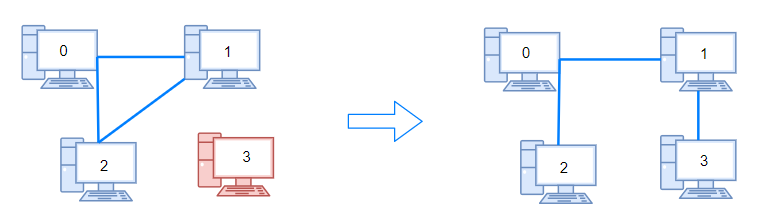
1 | Input: n = 4, connections = [[0,1],[0,2],[1,2]] |
方法一:dfs. 如果线够,就可以将所有的服务器相连,找到连通器的个数然后-1,就是需要的操作数。
1 | def makeConnected(self, n: int, connections: List[List[int]]) -> int: |
方法二:并查集。
1 | def makeConnected(self, n: int, connections: List[List[int]]) -> int: |
1489. Find Critical and Pseudo-Critical Edges in Minimum Spanning Tree
找到最小生成树的关建边和伪关建边。关建边指去掉这条边以后,路径和会增加。伪关建边,因为生成树图不唯一,指有的生成树图中有这条边,有的没有。还有一种是冗余边,指的是必须要去掉的边。分别返回关建边和伪关建边的索引。
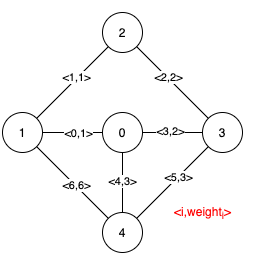
1 | Input: n = 5, edges = [[0,1,1],[1,2,1],[2,3,2],[0,3,2],[0,4,3],[3,4,3],[1,4,6]] |
方法一:Union-Find & Kruskal’s 算法。按照Kruskal算法,我们先将所有的边按权重排列。先将所有点边传入得到一个最小生成树的路径和S。再将每条边去掉剩下的边求路径和S1,如果大于S,则说明这是一条关建边;否则它可能是一条伪关建边或者冗余边。然后我们将这条边加到图中,生成一个路径和S2。如果S2和S1相等,则说明是伪关建边。
1 | def findCriticalAndPseudoCriticalEdges(self, n: int, edges: List[List[int]]) -> List[List[int]]: |
方法二:待定
1579. Remove Max Number of Edges to Keep Graph Fully Traversable
找到能使Alice,Bob任意节点都能遍历完整图的最多删除的边的条数。
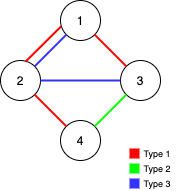
1 | Input: n = 4, edges = [[3,1,2],[3,2,3],[1,1,3],[1,2,4],[1,1,2],[2,3,4]] |
方法一:贪心+并查集。优先保留类型3的边。注意类型3的边也可能增加结果。
1 | class UF: |